Table of Contents
Feeling frustrated working with Date in Javascript? The Date API is complex and lacks almost any method respecting immutability. If you find it unconvincing, I’ll show you why. Despite months in Javascript starting from 0, days in a month start from 1. To create a new date for January, you would have to call new Date(2024, 0, 1). This can be confusing for beginners and overall, it’s not very intuitive. That’s just one of the countless reasons why the development community has created libraries like moment.js or date-fns to make working with Date easier. The good news is that we no longer need to rely on third-party libraries. Javascript’s Temporal API can completely replace them and address the issues we often encounter when working with Date.
Table of Contents
1. What Is The Temporal API?
The temporal API brings a new global object called Temporal to JavaScript that includes TONS of new methods and multiple new classes for handling a variety of date based concerns.. The goal of Temporal is to make working with dates/times easier and simpler for programmers. If you’ve ever encountered errors when trying to convert a string into a Date or complained that Date only supports the Gregorian calendar and not the Chinese lunar calendar or other calendar types, and even found it frustrating that Date are too easily mutable, now Temporal is the perfect solution for you.
2. Core Functionality of the API
Let’s try to get familiar with the API and see what are its core functionalities. A common example is getting the current date and time. This can be done in the following way:
Notice that we called toString on the return value. This is because Temporal returns an object that contains all of the metadata associated with the date, including:
So you can actually call all of the above properties right on the date to get whatever information you need. For example, the day or the month. But in fact, Temporal.Now has many more methods that you can call, including the following:
You can also construct new dates and times from variables, using:
3. Helper Methods
Add and subtract
With the Temporal API, all its data types have built-in methods supporting addition and subtraction, making the process of date and time calculations straightforward. The parameters for both functions are similar, except one performs the subtraction operator and the other performs addition.
To perform this, we only need to pass the attributes we want to modify:
Another nice thing about these functions is they automatically deal with overflow. For example if you try to add 1 month to the date January 31st that would result in the date February 31st which doesn’t exist. By default, these results will be constrained to the nearest valid date, so it will return February 28th (or February 29th in a leap year). You can disable this behavior, though with a second options argument.
Since and until
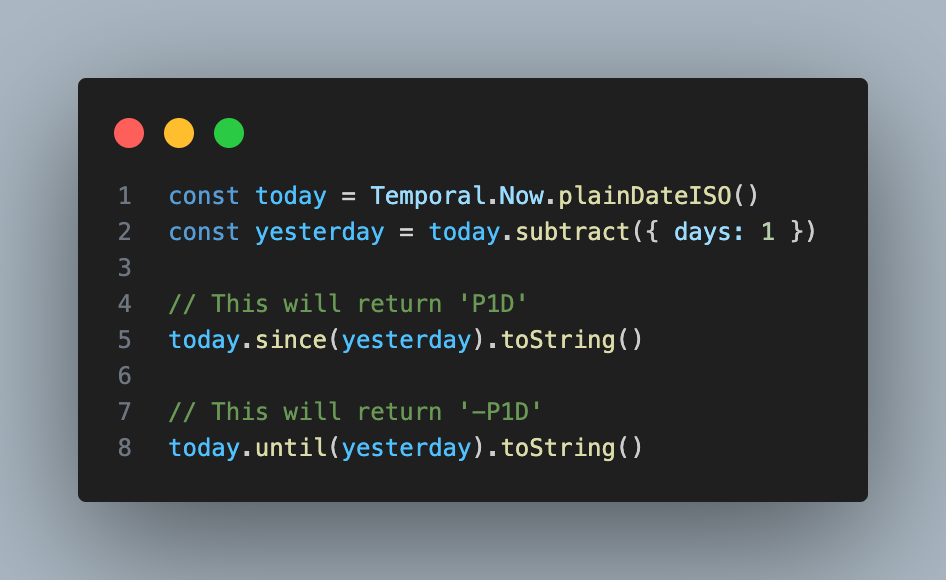
Also, you can pass an options argument to these methods to really fine tune how you want the duration to be calculated.
If you specify the largestUnit then the duration will be specified using that unit as the largest value instead of the default value:
If you specify the smallestUnit then the duration will be specified using that unit as the smallest value instead of the default value. This could result in rounding which can be further customized with the roundingIncrement and roundingMode options:
Equal
The Temporal API also has the ability to compare two different dates. For example, if you want to see if two dates are equal, you can do the following:
This is needed since technically any comparison done with ==
or ===
will be false unless the two objects are the same instance.
Compare
Dates can also be sorted through the Temporal API. Suppose we have 5 different dates in random order. The compare method of PlainDate can help us sort the dates in ascending order:
With
This is one of my favorite helper methods since it covers a huge weak point in JavaScript dates. The with method takes in an object of fields to overwrite on the current date object.
Also, something important to know about this method is that it does not actually change the temporal date object it is called on. It instead returns a new temporal date object with the changes applied to it.
Round
If you want to round a temporal date to a specific unit this method is perfect:
If you want to modify how the rounding is performed you can instead pass an object that takes smallestUnit, roundingIncrement, and roundingMode:
Total
Last but not least, and equally important, you can also use the Temporal API to calculate durations through the use of Temporal.Duration:
Here, I’m constructing a Duration object with 2 hours and 15 minutes and want to know the total number of minutes. Of course, you can customize the number of hours, minutes, and seconds as needed.
4. Conclusion
Dates in Javascript indeed come with many issues, but with the Temporal API, every time you work with dates, you will feel fantastic.
Reference: Temporal Date API Ultimate Guide, First Look at the Temporal API in JavaScript
Sona